2021. 4. 12. 16:50ㆍProjects/React.js로 영화 웹 서비스 만들기
#3.2 Component Life Cycle
🍑 이번 시간에는 리액트 컴포넌트의 life cycle 메소드에 대해서 알아보겠다.
현재 우리는 리액트 컴포넌트에서 제공하는 메소드 중에서 render 메소드만 사용하고 있다.
그런데 사실 리액트 컴포넌트는 단순히 render 말고 더 많은 걸 가지고 있다.
이들은 life cycle 메소드를 가지는데, life cycle 메소드는 기본적으로 리액트가 컴포넌트를 생성하는 그리고 없애는 방법이다.
컴포넌트가 생성될 때 render 전에 호출되는 몇 가지 function이 있다.
컴포넌트가 render 된 후 호출되는 function 들이 존재한다.
예를 들면 우리가 add 함수를 통해 +1 +1 +1을 할 때 , 컴포넌트가 업데이트 될 때 호출되는 다른 함수가 있다는 것이다. 전부다 살펴보지는 않을 것이고 우리가 필요로 하는 가장 중요한 것을 살펴볼 것이다.
🍑 Life cycle 메소드의 종류
Life Cycle 메소드 종류에는 3가지가 있다.
1) Mounting - 컴포넌트의 생성
2) Updating - 컴포넌트의 업데이트
3) Unmounting - 컴포넌트의 소멸
(컴포넌트가 죽는 것, 언제 컴포넌트가 죽지? 페이지를 바꿀 때, state를 사용해서 컴포넌트를 교체하기도 하고 .. 컴포넌트가 죽는데에는 다양한 방법이 있다. )
🍓 1. Mounting
* constructor() : constructor는 리액트에서 온 것이 아니라 자바스크립트에서 클래스를 만들 때 호출되는 것이다.
* render()
* componentDidMount() : 컴포넌트가 생성되고 호출된다.
순서: constructor -> render -> componentDidMount
- 컴포넌트가 mount 될 때, 컴포넌트가 screen에 표시될 때, 컴포넌트가 너의 웹사이트에 갈 때 constructor를 호출한다. 그리고 나서 render 이다. 이 후 컴포넌트가 render된 후 componentDidMount()가 실행된다. 기본적으로 componentDidMount는 우리에게 알려준다. "이봐 이 컴포넌트는 처음 render 됐어"
componentDidMount 사용해보기
class App extends React.Component{
state = {
count: 0
};
add = () => {
this.setState(current => ({count: current.count + 1}));
};
minus = () => {
this.setState(current => ({count: current.count - 1}));
}
componentDidMount(){
console.log("component rendered");
}
render(){
console.log("I'm rendering");
return (
<div>
<h1>The number is : {this.state.count}</h1>
<button onClick={this.add}>Add</button>
<button onClick={this.minus}>Minus</button>
</div>
);
}
}
이렇게 작성하고 콘솔을 확인해보면
I'm rendering
component rendered
라고 뜨는 것을 확인해볼 수 있다. 즉 componentDidMount()는 render() 후에 실행되는 것이다.
🍓2. Updating
* render()
* componentDidUpdate() : 컴포넌트가 업데이트 되고 호출된다.
componentDidUpdate() 사용해보기
App class안에 다음과 같이 작성해본다.
componentDidUpdate(){
console.log("I just updated");
}
render(){
console.log("I'm rendering");
return (
<div>
<h1>The number is : {this.state.count}</h1>
<button onClick={this.add}>Add</button>
<button onClick={this.minus}>Minus</button>
</div>
);
}
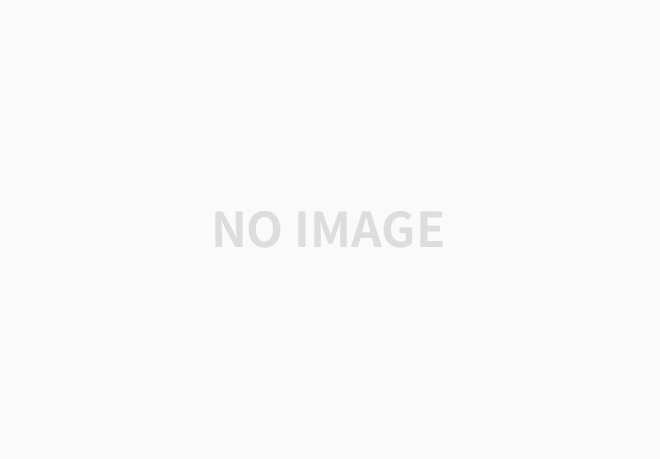
그리고 실행해보면 처음에 컴포넌트가 mount되면서 render() -> componentDidMount()가 실행되었고
add 버튼을 클릭해서 업데이트를 시키면 render()를 한 후 componentDidUpdate()가 실행되는 것을 볼 수가 있다.
🍓3. Unmounting
* componentWillUnmount
컴포넌트가 소멸될 때 호출된다. (다른 페이지로 간다거나)
'Projects > React.js로 영화 웹 서비스 만들기' 카테고리의 다른 글
#4.0 Fetching Movies from API (0) | 2021.04.14 |
---|---|
#3.3 Planning the Movie Component (0) | 2021.04.12 |
#3.1 All you need to know about State (0) | 2021.04.12 |
#3.0 Class Components and State (0) | 2021.04.12 |
#2.4 Protection with PropTypes (0) | 2021.04.12 |